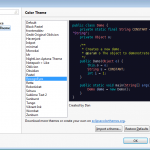
I spend quite a lot of time using Eclipse and the white background is not particularly helpful for my eyes. I wanted a Java theme which is more relaxing for my eyes (e.g. with black background). The solution is Continue reading
I spend quite a lot of time using Eclipse and the white background is not particularly helpful for my eyes. I wanted a Java theme which is more relaxing for my eyes (e.g. with black background). The solution is Continue reading
By default on Windows Eclipse autocomplete shortcut is: Ctrl+Space. In my Mac laptop I use this combination for the operating system’s spotlight quick search. As a result, I wanted to change the default autocomplete shortcut. What you need to do is:
1. Go to Window->Preferences (Eclipse -> Preferences on Mac).
2. In the new window that pops up, go to “General -> Keys”
3. Find the “Content Assist” command and change it to whatever you like (in my case I chose Cmd+Space).
4. Don’t forget to click on “Save” on this pop up window!
I recently wanted to install Visual Editor on Eclipse Helios. Apparently there is an nice way, which works like a charm for me. For more details you can have a look here: http://sourceforge.jp/projects/tmdmaker/wiki/VisualEditor1.4.0ForHelios
From time to time I develop something on Eclipse. Apart from the usual Eclipse operations (build and test) that I do with the Eclipse Ant and JUnit plugins (they ship with Eclipse for Java), I also wanted to carry out some dependency analysis on existing code. Of course JDepend is the obvious option, but I found a nice article from IBM that suggests some additional plugins that help the coder carry out day to day tasks such as Complexity monitoring and Coding standard analysis.
The following is a summary of the plugins proposed by the article. Of course all of them are opensource/free.
Tool | Purpose | URL for Eclipse plugin |
CheckStyle | Coding standard analysis | http://eclipse-cs.sourceforge.net/update/ |
Coverlipse | Test code coverage | http://coverlipse.sf.net/update |
CPD | Copy/Paste detection | http://pmd.sourceforge.net/eclipse/ |
JDepend | Package dependency analysis | http://andrei.gmxhome.de/eclipse/ |
Metrics | Complexity monitoring | http://metrics.sourceforge.net/update |
The full IBM article can be found here: http://www.ibm.com/developerworks/java/library/j-ap01117/
I recently installed eclipse 3.3 and Java 1.6. Today I tried to use SiTra (Simple Transformations in Java) but when I tried to compile it Java started complaining on the following methods of the SimpleTransformerImpl.java:
public Object transform(Object object) { return transform(Rule.class, object); } public List<? extends Object> transformAll(List<? extends Object> sourceObjects) { return transformAll(Rule.class, sourceObjects); }
The error was something about java inconvertible types. I don’t know if it is the new version of eclipse or Java 1.6. Looks like for some reason the compiler doesn’t recognise that Rule.class is of type Class<? extends Rule<S, T>>, so as to call the public <S, T> T transform(Class<? extends Rule<S, T>> ruleType, S source)
A simple solution is to ct the Rule.class expression to Class (it sounds weird but it worked).
So the transform and transformAll methods become:
public Object transform(Object object) { Class r = Rule.class; return transform(r, object); } public List<? extends Object> transformAll(List<? extends Object> sourceObjects){ Class r = Rule.class; return transformAll(r, sourceObjects); }